A tutorial for those new to Perl but not to Programming: Part 0
Introduction
When you don't have much to blog about, you start trying to think about things you already know. Well... I know Perl, and I have some friends who would like to learn it, or at least be comfortable with it. I'll present this tutorial in progressively more advanced parts, showingcasing a little at a time the high and low points of Perl and how to use it.
What is Perl?
Perl is lots of things to lots of people, but to me - and many other people - it's a swiss army knife language (if you will). When you pull a bunch of random data out of an excel spreadsheet and you need to do some stuff with it real quick (like, maybe, remove invalid email addresses and then send a mass message), you don't compile a huge C application and you don't need to power up your Java VM. You just write a few lines of Perl.
Perl has had other purposes, too. Sometimes referred to as the glue that holds the web together, Perl has been used extensively throughout the years in CGI scripting, although it is my believe (but not quotable experience) that it is falling out of use with the evolution of the internet into a far mor
e interactive and m
ultimedia-oriented experience... although I also know that there is still plenty, plenty, plenty of Perl out there.
Perl is also great for just hacking things together and making things work. If you had a round peg, a square hole, and a pocketknife, Perl would be that pocketknife. Observe this comic from xkcd.com (released under Creative Commons; kudos to Randall Munroe for his amazing webcomic):
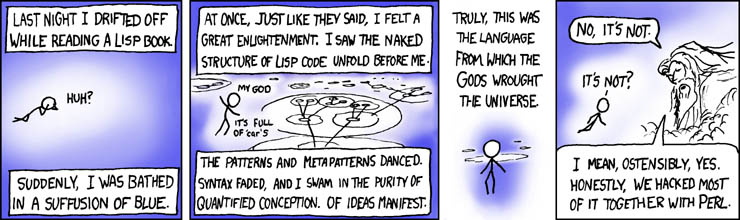
(Click for larger view.)
Anyway, that's about what Perl is.
Jumping Right Into It
No tutorial would be complete without...
#!/usr/bin/perluse warnings;use strict;print "Hello, world!";
There's our familiar Hello, world! program. The first three lines are unnecessary, generally. You could write the program as simply print "Hello, world!";, execute perl
Line 1: #!/usr/bin/perl
This line is often called a shebang, and is common to many Unix scripts. In fact, if you go find a bash script on your computer, chances are that the first line is something like #!/bin/sh. That's because when you run chmod +x script.pl to turn your script into an executable file, it looks as that first line to determine what needs to be called to run it.
Of course, if you're running Windows, none of this will make any sense. If, for some reason, you are in a Windows system, this first line won't help you out much. Just run your scripts using the perl syntax and everything will be okay. Depending on your Perl interpreter (I know that ActivePerl works this way), you could also just double-click on your script in Explorer and it should run. Be wary that if you're script isn't actually doing anything functional - for example, if it's just the Hello, World! script above - then you probably won't see anything happen besides a terminal appear for a few milliseconds and disappear again, because the terminal's process will die along with the Perl script.
But be warned that if you're running Windows, you probably won't be able to use a lot of the things in this tutorial. Go use Visual Basic or something.
Anyway...
Line 2/3: use warnings; use strict;
The use of the warnings module alerts us when we do silly things. It won't stop our program from running, but it might let me know if, say, I declared a variable I never used. Sounds stupid? Not really. Perl is extremely nice to its user and tries to do exactly as it is told... which can be a problem. Say I have the variable $rest = 5; and then later on I mistype it: for ($x=0; $x<$breast; $x++).... Normally, an interpreter/compiler would catch this Freudian slip (perhaps you had some inappropriate browser windows open while coding), but Perl will do its best to do exactly as you say, so it'll instantiate $breast for you with the default value of 0. This will not function correctly. Using warnings will probably give you a little wake up call if you knew this variable was supposed to be in use. This is only an example, though; the warnings module is extensive and highly useful for debugging. The use strict flag makes you declare all variables with a some sort of scope modifier, which we'll talk about later, but it is important to note that, unlike many languages, a variable declared in any scope will, by default, become global. This often causes trouble if not handled with care.
If you can't figure out line 4, you probably shouldn't be reading a tutorial for people who already have some programming experience. In fact, you should probably understand line 4 whether you have programming experience or not.
Variables
Watch this:
$variable = 5;$variable = "Hello, Janet!";$variable = \$referenced_var$variable = \&referenced_subroutie$variable = -234.3$variable = 0x0000003f;$variable = "Some other data type!";print $variable; # prints out "Some other data type!"... and yes, this is a valid program.# By the way, this is a comment!
Perl has a what's called a dynamic typing system. Several other languages have this as well (Ruby and Python, to name two) but it isn't typical of what you see in C, C++, or Java. Whereas in those languages you must declare a variable's data type (either beforehand or on-the-fly), Perl variables can hold any type of data, any time... the only restriction is on memory (which, these days, is considerable).
Note: Perl 6 (which will be similar but also considerably different from Perl 5 and earlier) will have an exciting new hybrid typing system which allows you to assign a value if you want to. For example, you could write the traditional, perlish: $variable = 5;, but you can also write the sometimes useful and more C-ish: int $variable = 5;, which will treat $variable just like C would.
More to come later!
No comments:
Post a Comment